Built for beauty
and speed
Cross-platform MIT-licensed Desktop GUI framework for C, C++, Python and Rust, using the Mozilla WebRender rendering engine
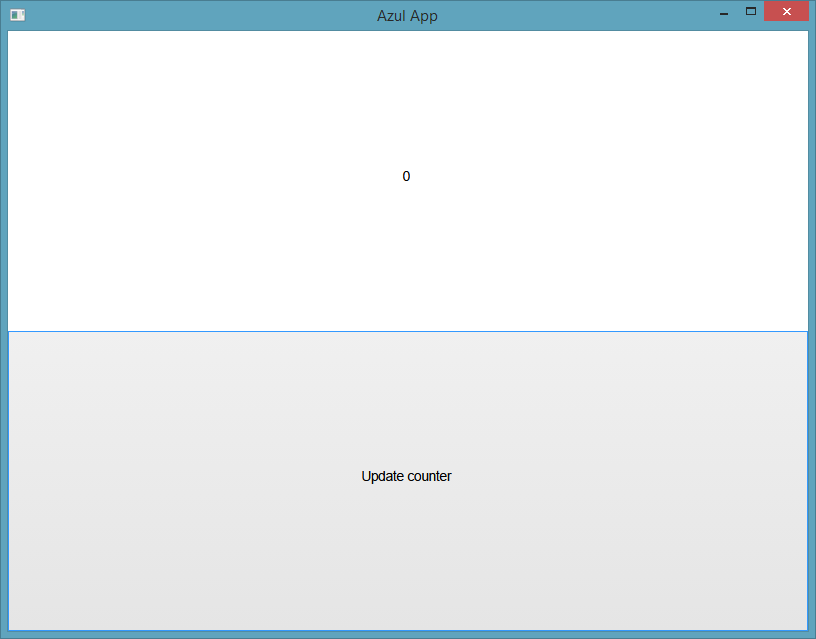
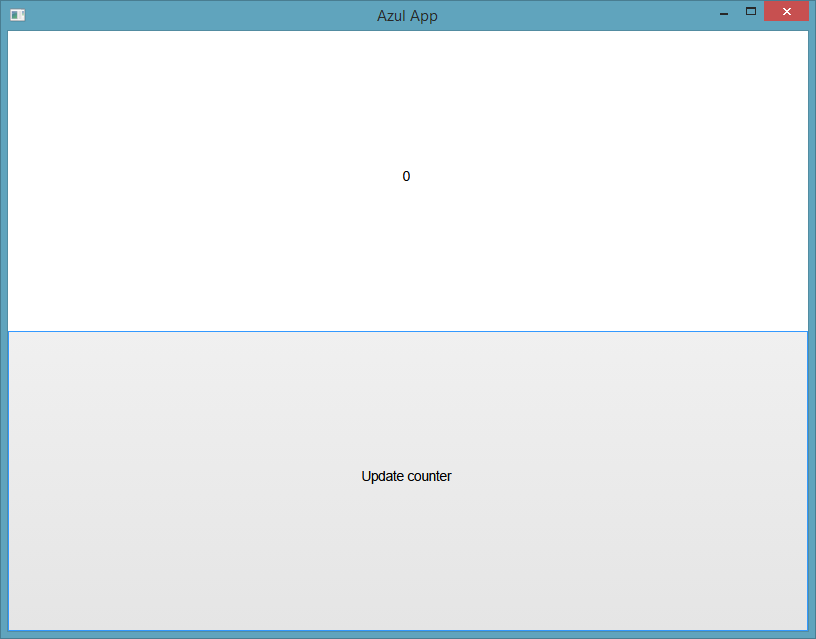
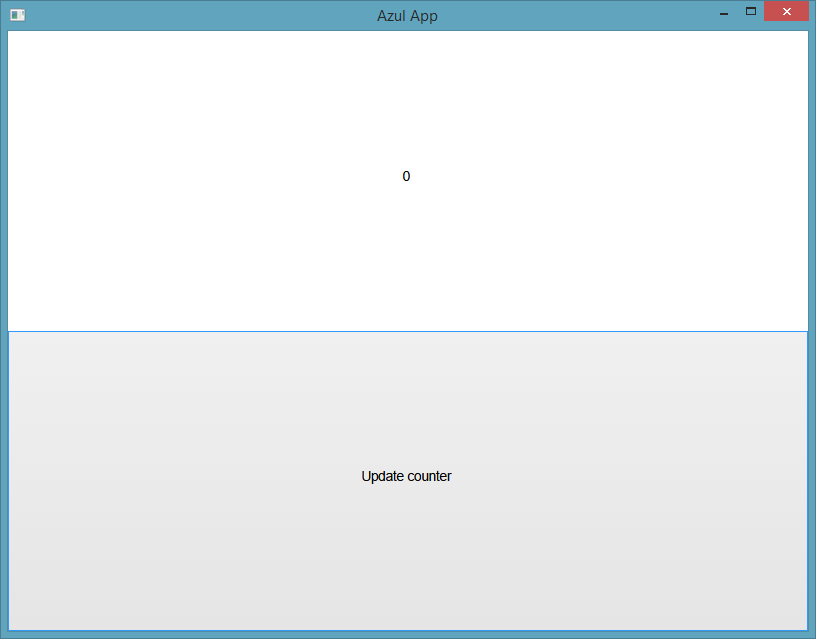
Azul composes objects in a DOM hierarchy with function pointer callbacks attached to nodes.
The DOM tree only gets re-rendered when any callback returns RefreshDom
: in difference to IMGUI, this
does not happen on every frame. The DOM tree represents valid XHTML and can be styled with CSS.
from azul import *
class DataModel:
def __init__(self, counter):
self.counter = counter
# model -> view
def my_layout_func(data, info):
label = Dom.text("{}".format(data.counter))
label.set_inline_style("font-size: 50px")
button = Button("Update counter")
button.set_on_click(data, my_on_click)
button = button.dom()
button.set_inline_style("flex-grow:1")
root = Dom.body()
root.add_child(label)
root.add_child(button)
return root.style(Css.empty())
# model <- view
def my_on_click(data, info):
data.counter += 1
return Update.RefreshDom
model = DataModel(5)
app = App(model, AppConfig(LayoutSolver.Default))
app.run(WindowCreateOptions(my_layout_func))
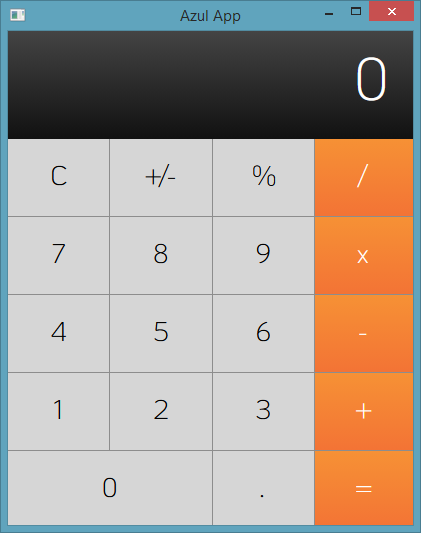
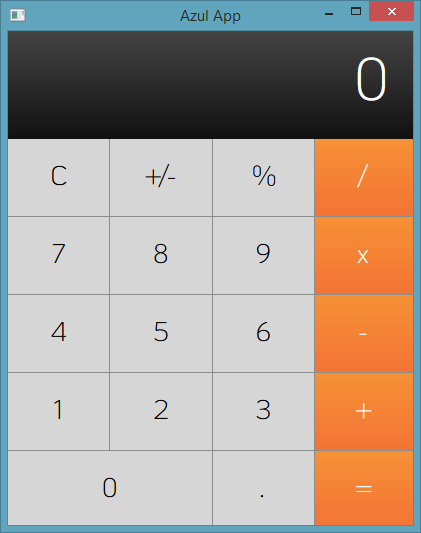
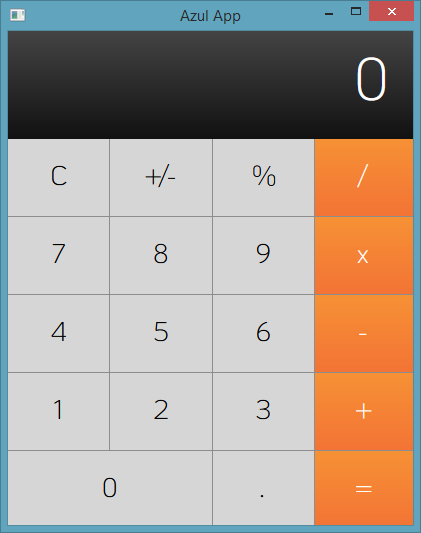
The UI can be constructed in various ways: serialized to / from a HTML file or composed via functions. Your application data never has to hold any references to UI objects, making Azul very non-intrusive.
from azul import *
def main():
pass
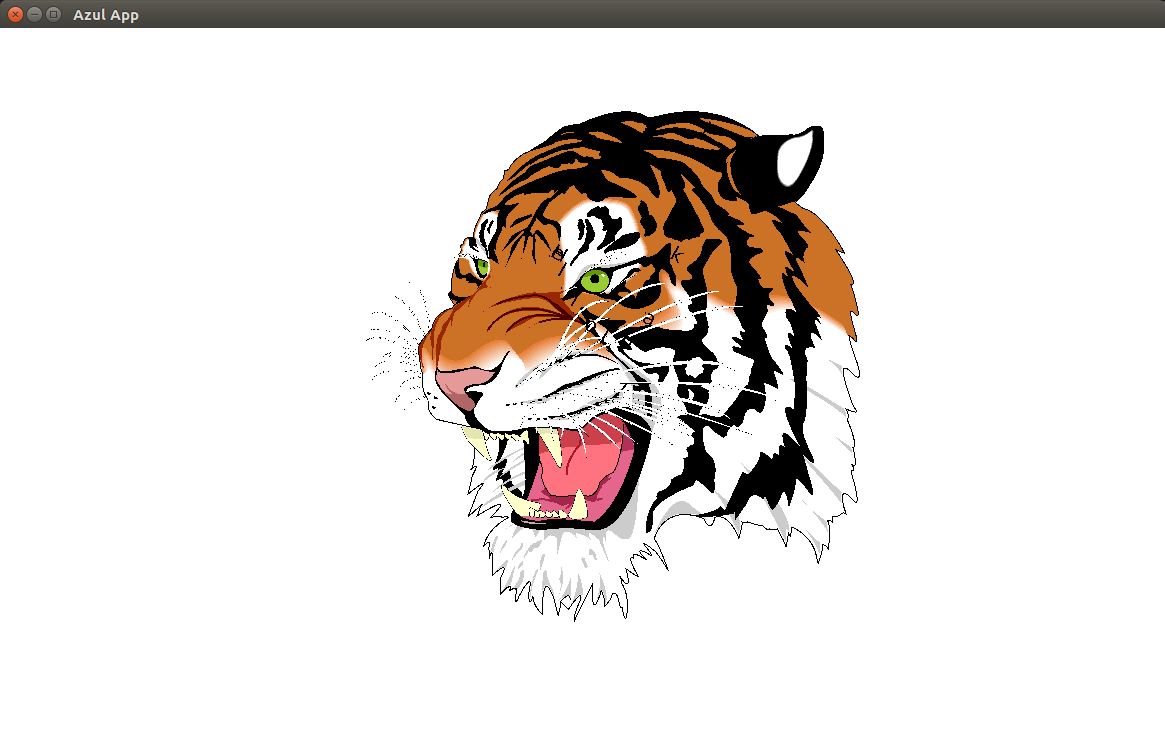
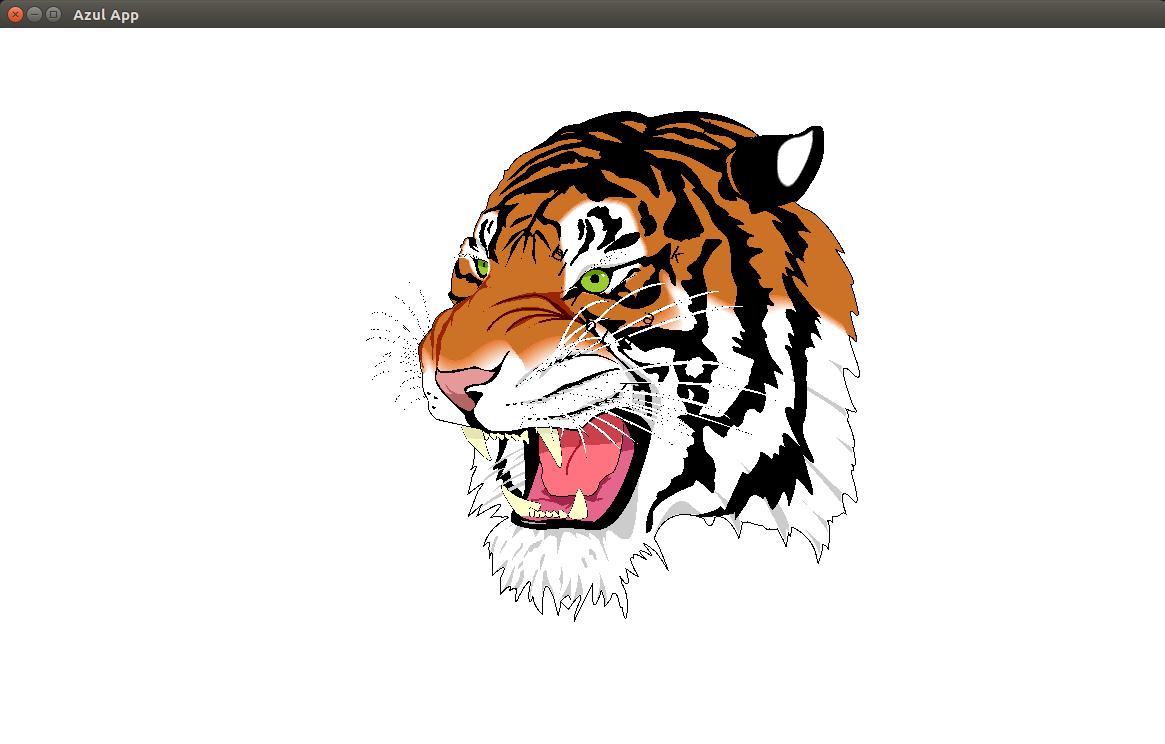
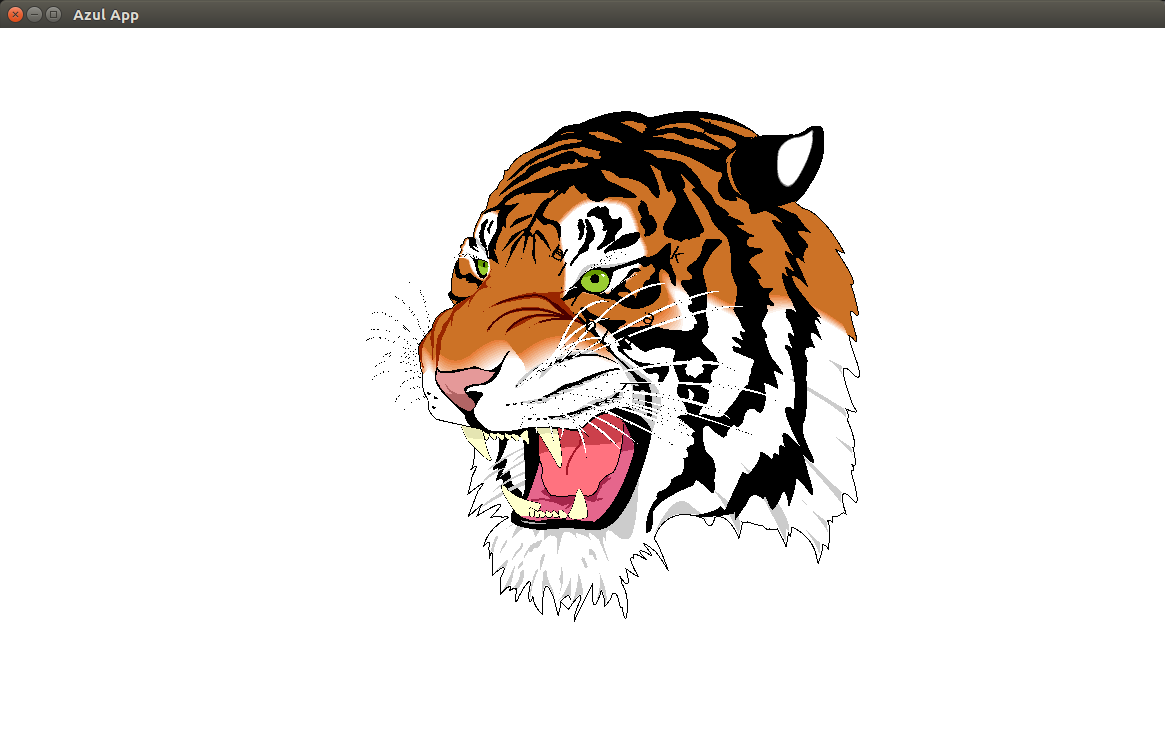
The layout solver (azul-layout) is a CSS2.1 / XHTML4 compliant solver, which is completely detached from a renderer. Thanks to the MIT license, you can embed a full HTML layout solver in your custom application, which handles font shaping and layout for you.
from azul import *
def main():
pass
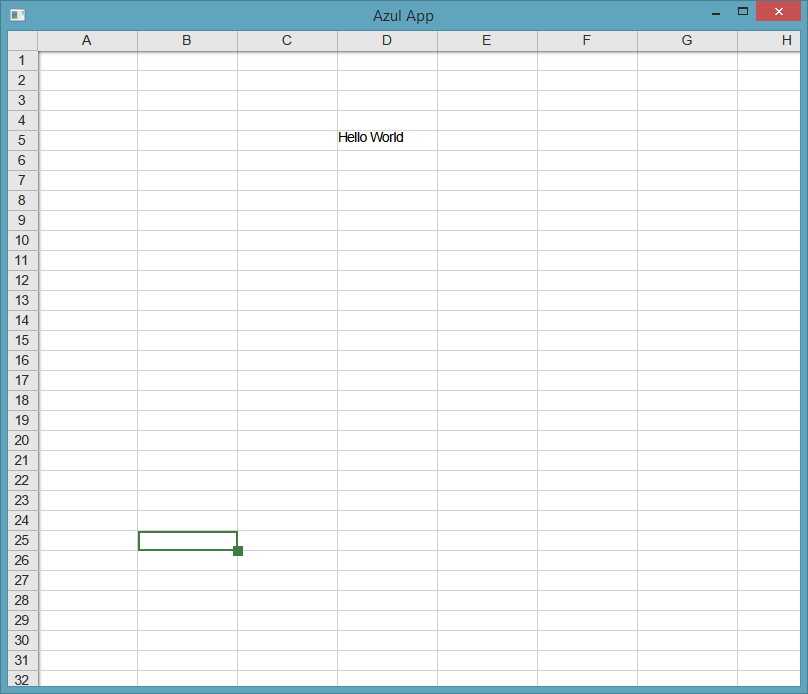
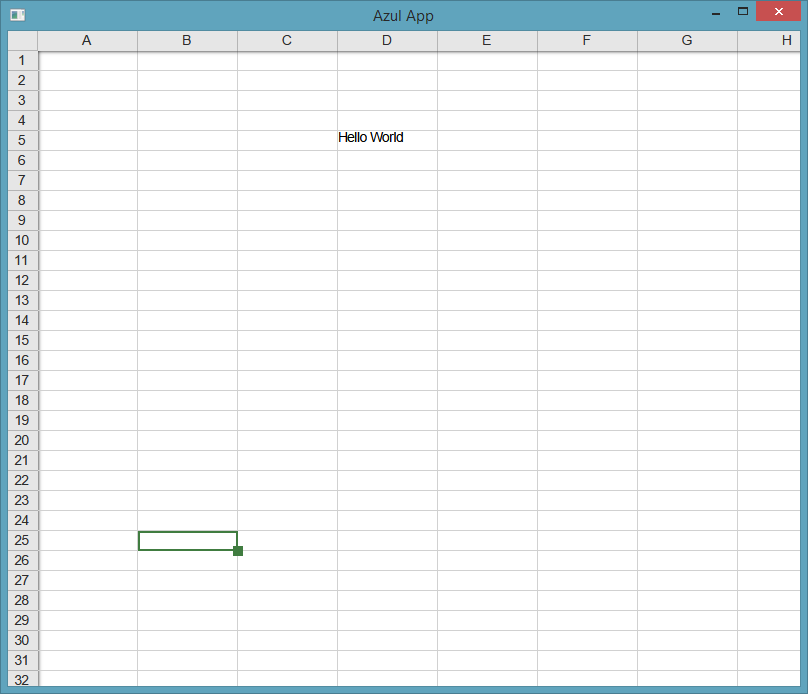
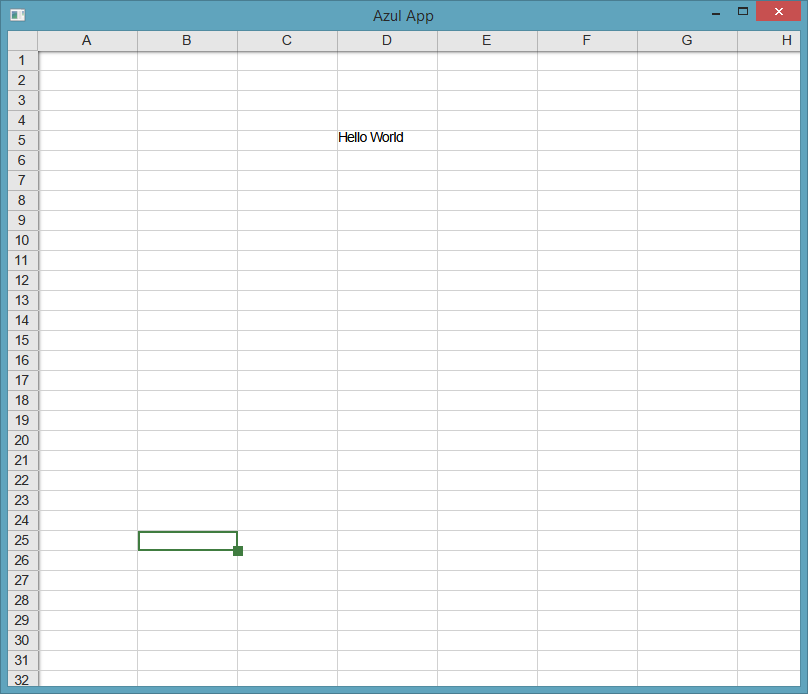
For extremely large or sparse datasets, Azul can render only parts of a DOM and automatically trigger re-rendering when the user scrolls.
from azul import *
def main():
pass
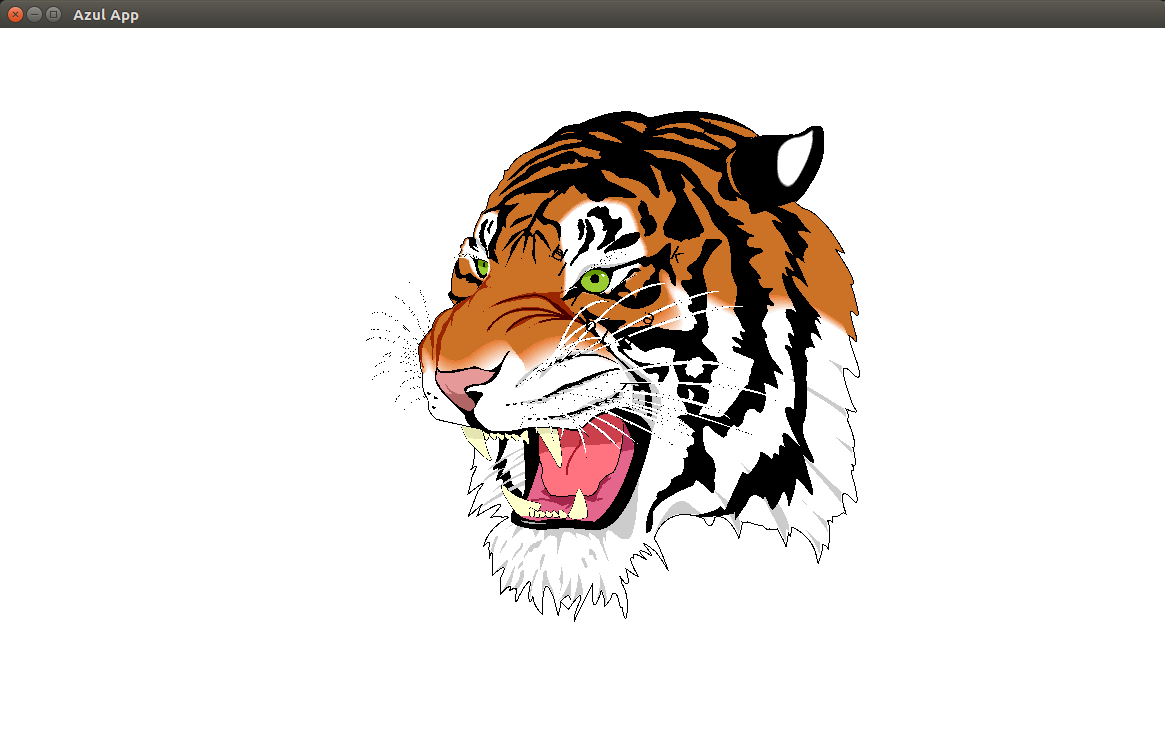
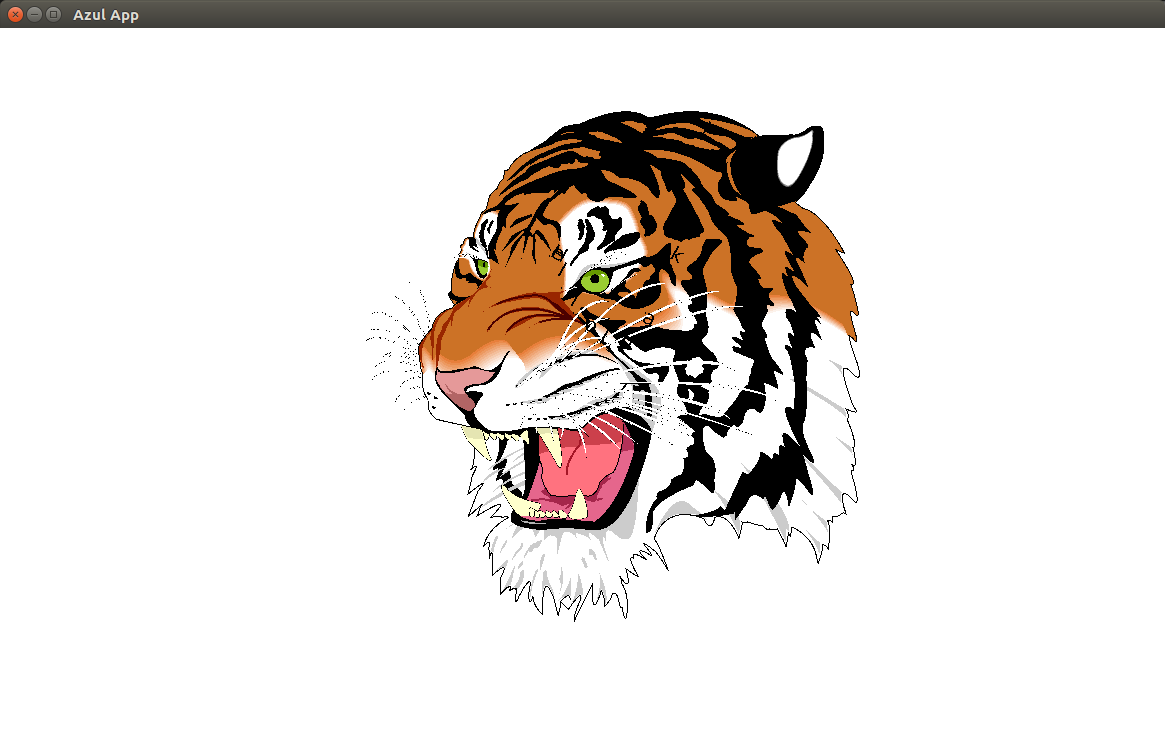
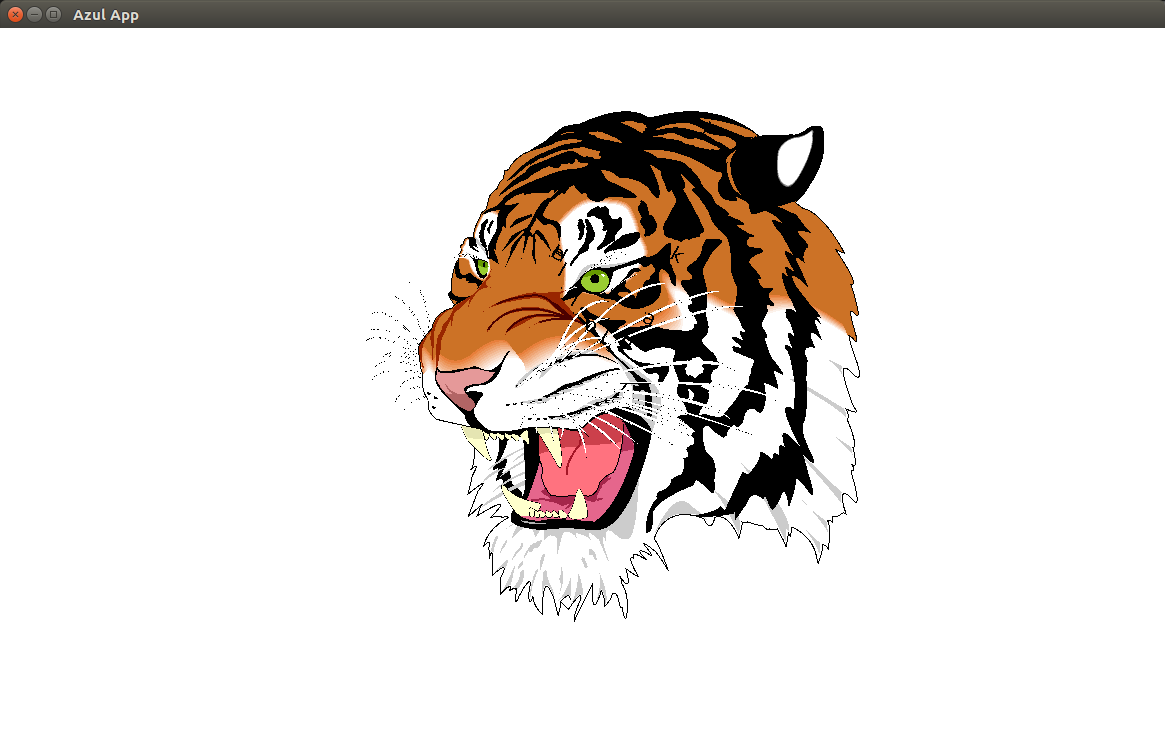
Azul exposes Rust utilities for image decoding and encoding, SVG rendering and parsing as well as font loading to C, C++ and Python applications.
from azul import *
def main():
pass